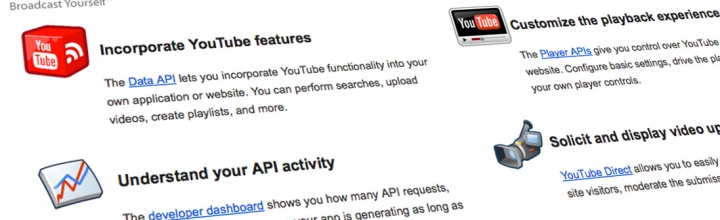
This is a class I’ve used several times and it demonstrates some of the useful data endpoints offered by YouTube.
Often I will have to ask users or a web service for YouTube videos, and these may come in the form of share URLs, embed code or video URLs. This class allows you to extract the video ID from each of these sources, which can be saved separately and used to either retrieve video data or embed the video in a page at a later date.
<?php /** * Simple class to extract YouTube Video ID and display video details * * @author Peter Smith - sandjam.co.uk * @version 1.0 10-9-12 */ class YouTube { public function __construct(){ } /** * Extract the video id from a YouTube URL or share code * * @param $str:String - URL or share code from YouTube * @return $vid:String/False */ public function getVideoId($str){ // get video id from url in the format http://anything/videoid preg_match("/(.*\/)([0-9a-zA-Z_-]*)$/i", $str, $matches); if (isset($matches[2]) && $matches[2]!='') { $vid = $matches[2]; } // get video id from url in the format http://anything/watch?v=videoid&anything preg_match("/(.*\/)watch\?v\=([0-9a-zA-Z_-]*)\&?.*$/i", $str, $matches); if (isset($matches[2]) && $matches[2]!='') { $vid = $matches[2]; } // get video id from embed code in the format <iframe width="xxx" height="xxx" src="http://www.youtube.com/embed/videoid" frameborder="0" allowfullscreen></iframe> preg_match("/iframe.*\/embed\/(.*)\"/iU", $str, $matches); if (isset($matches[1]) && $matches[1]!='') { $vid = $matches[1]; } if (!isset($vid)) { return false; } $vid = trim($vid); return $vid; } /** * Get the video details from YouYube data api * * @param $vid:String - YouTube Video Id * @return $details:Array */ public function getVideoDetails($vid){ // attempt to load video to check it exists. This returns 404 if any errors with the videoid $response = @file_get_contents('http://gdata.youtube.com/feeds/api/videos/'.$vid); // response is not xml (error code) if (substr($response, 0, 5) !='<?xml') { echo "$vid - This does not seem to be a valid YouTube video"; return false; } // assume thumbnail image exists in the usual location $image = "http://i.ytimg.com/vi/$vid/0.jpg"; // parse video details xml $videoXml = new SimpleXMLElement($response); $title = $videoXml->title; $details = array( 'title' => (string) $videoXml->title, 'description' => (string)$videoXml->content, 'author' => (string)$videoXml->author->name, 'author_url' => (string)$videoXml->author->uri, 'date' => (string)$videoXml->published, 'image' => (string)$image ); return $details; } /** * Compose the embed html code from a video id * * @param $vid:String, [$width:Int, $height:Int] * @return $html:String */ public function getEmbedCode($vid, $width=600, $height=400){ $html = '<iframe frameborder="0" width="'.$width.'" height="'.$height.'" allowfullscreen="" src="http://www.youtube.com/embed/'.$vid.'?fs=1&feature=oembed&wmode=opaque"></iframe>'; return $html; } } ?>